27일차
Prototype 상속
1. 프로토타입 체인을 이용한 상속
- 자바스크립트 객체는 속성을 저장하는 동적인 ‘가방’과 프로토타입 객체 에 대한 링크를 가진다.
- 객체의 어떤 속성에 접근하려 할 때 그 객체 자체 속성 뿐만 아니라 객체의 프로토타입, 그 프로토타입의 포로토타입 등 프로토타입 체인의 끝까지 이를 떄 까지 그 속성을 탐색한다.
2. class 상속
- 클래스를 생성할 때 다른 클래스를 상속 받을 수 있는데, 새로 만들어지는 클래스가 자식클래스, 상속하는 클래스가 부모 클래스가 된다.
- 자바스크립트에서 상속은
extends
를 사용한다.
class Person{
constructor(name, first, second){
this.name = name;
this.first = first;
this.second = second;
}
sum() {
return this.first + this.second
}
}
class PersonPlus extends Person{
avg(){
return (this. first+ this.second) /2;
}
}
let kim = new PersonPlus('kim',10,20)
console.log('kim.sum()', kim.sum()) // kim.sum() 30
console.log('kim.avg()',kim.avg()) // kim.avg() 15
- PersonPlus 에 sum() 메소드가 없음에도 불구하고 kim 객체에 sum() 을 호출하니 에러없이 값이 나왔다.
- 이는 PersonPlus 의 부모클래스 Person 의 sum() 메서드를 사용한 것이다.
- 부모클래스와 같은 함수명을 새로 정의하는 것을 오버라이딩이라고 한다.
3. super
- 클래스를 생성할 떄, 부모클래스의 기능을 활용해서 메소드나 변수를 선언하고 싶을떄 사용
class Person{ constructor(name, first, second) { this.name = name; this.first = first; this.second = second; } sum(){ return this.first + this.second; } } class PersonPlus extends Person{ constructor(name, first, second, third) { super(name, first, second) //Person constructor에 선언된 name, first, second 변수 가져다 사용, 정의되지 않은 third 는 새로 선언 this.third = third } sum(){ return super.sum() + this.third; } avg(){ return (super.sum() + this.third) /3; } } let kim = new PersonPlus('kim, 10, 20, 30) console.log('kim.sum()', kim.sum()) // kim.sum() 60
4. proto, constructor, prototype
- 자바스크립트 함수는 객체이기 때문에 property를 가질 수 있다.
function Person(name, first,second) { this.name = name; this.first = first; this.second = second }
- 함수에 해당되는 Person이라는 객체가 생긴다. 동시에 Person’s prototype 이라는 객체가 하나 더생긴다
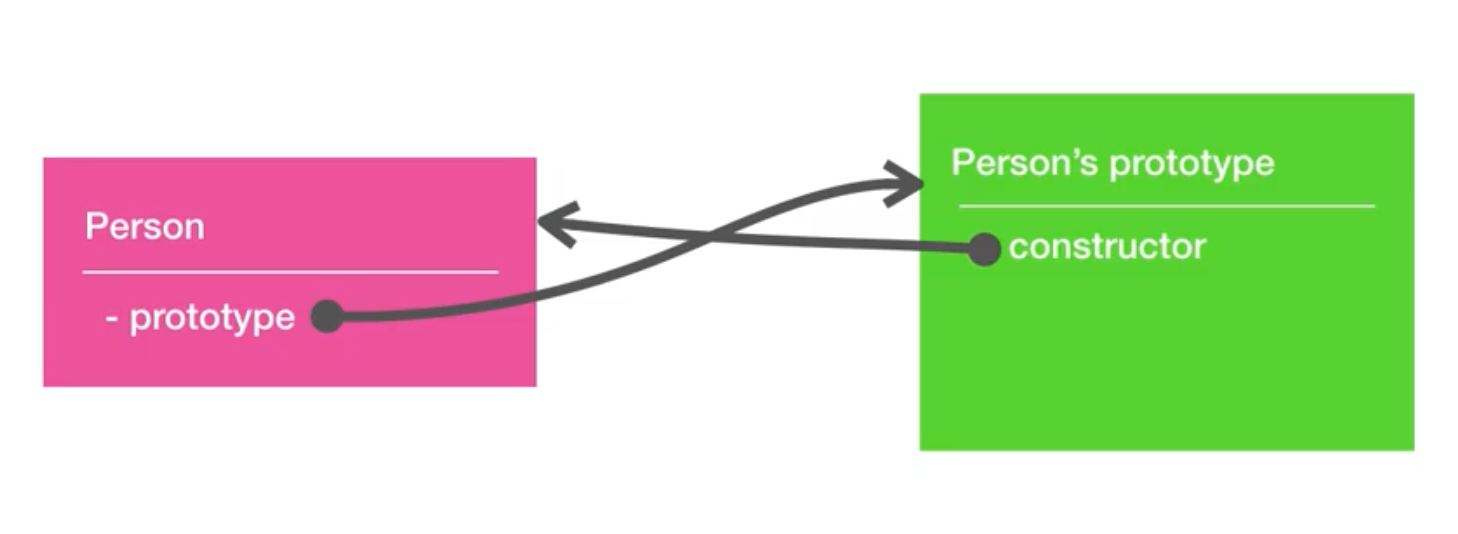
Person.prototype.sum = function(){}
let kim = new Person('kim, 10, 20)
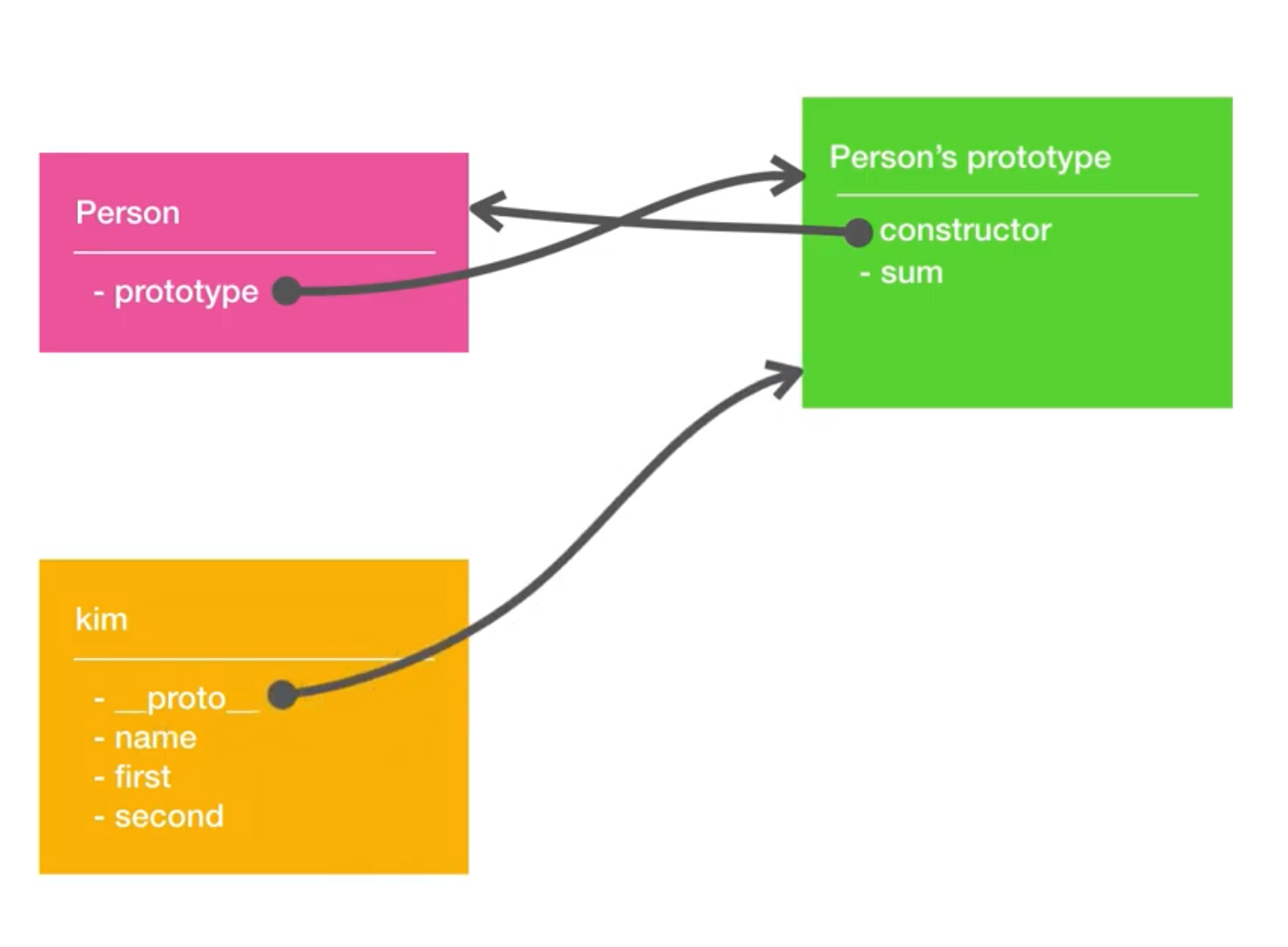
- kim의
__proto__
는 Person’s prototype 를 가리키게 된다 - kim에는 sum() 이라는 메소드가 없기떄문에
__proto__
을 통해 Person’s prototype 에 sum()이 있는지 확인한다 - 만약에 sum()이 없으면 Person’s prototype 의
__proto__
을 통해 상위 prototoype 에 해당 메소드가 있는지 확인한다. - 객체가 직접적으로 않은 데이터를 사용할때, 어떤 데이터를 근거로 어디서 그 객체가 가지고 있지 않은 sum()을 찾아내는가가 핵심!